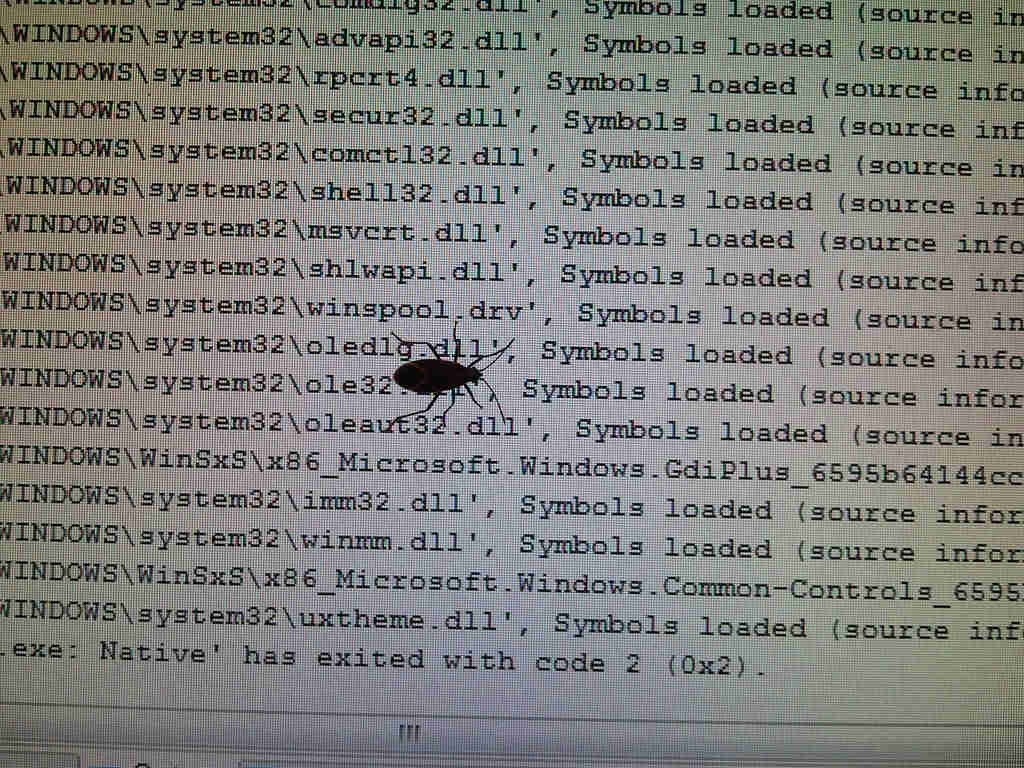
Debugging By Michael Mol.
Top 5 time wasters yet simple programming mistakes
People says if you have no errors or mistakes that means that you are doing nothing. Well it seems that is true, but that does not have to mean you have to commit the same mistakes that some else have committed before. Here are my top 5 time wasters yet simple mistakes.
Comparison errors
As you may know, the programming languages have many operators that can perform specific operations as assignment, comparisons, arithmetic operations and some others. All these operators usually return something. In the case of the assignment the value assigned is the value returned. In the case of the comparisons a boolean value is returned. That happens with most of programming languages.
var thing = "thing"; //"thing" is returned
var thing = 0<1; //"1" (true) is returned
In all the programming languages similar to C. The equal comparison operator is based on a double equal symbol ==
and the assignment operator is based on a single equal symbol =
. As you see both operator are pretty similar and you might commit a mistake here. but what will happen exactly?. The if sentence will evaluate whether the sentence between brackets is false or not. if is not false it will execute the first code block. If is false it will evaluate the second code block. However if we put an assignment operator instead of a comparison it will return the value assigned all the time silently.
//"thing" (a string) is returned
//and will be interpreted as true,
//making the program do other things (silent error)
if(thing = "thing"){
// this code will be executed all the time
}else{
// this code will never be executed
}
Solution
The first thing to do here is being careful. The second is a solution based on a coding standard (that I do not remember very well, being honest) that forces to place the value to compare before the variable (in case of comparisons between a variable and a string). So if you forget to place a double equal symbol it would throw an error immediately.
// this error will not be silent
// and, at least, you will get noticed somehow
if("" == thing){
//code
}
however this will not work for comparisons between two variables, so you need to be careful anyway.
“Copy/Paste” errors
Being honest, who has never copied a code from internet before?. I do it sometimes. At least, when reading some manuals. however there might be a chance that the inertia along with the desperation to complete something make you do mistakes. Especially when copy/pasting code from another source. Let us review the following three blocks of code. The two firsts are completely different and the third is the result of copy/pasting;
//first block
$.ajax({
type: "POST",
url: "test.php"
}).done(function( msg ) {
alert("data: "+msg);
});
//second block
$.ajax({
type: "POST",
url: "test.php"
}).done(function( html ) {
$("#element").append(html);
});
//copy/paste result, it will not work
$.ajax({
type: "POST",
url: "test.php"
}).done(function( msg ) {
// first, let's suppose the #element element exists
$("#element").append(html); // as you notice there is an unmodified variable name
//that it had to be changed therefore the code will not work
});
The third code is supposed to perform an AJAX request to a server and show the result in a HTML element. As you may notice, there is a copied line that was partially modified while copying it here, there is a little detail that should have been modified and is making the program to throw an error. Depending on your desperation and inertia, this mistake may take you a few valuable minutes (or hours) trying to solve this error. Some other mistakes as this may take you more time. Additionally, depending on the license (and other things such as the source) of the code copied. It is very likely that you are committing an infringement of the law and other people (copy)rights.
Solution
There is only one real solution, “READ”. If you do not read you are not really paying attention to the things you are doing. Something that is really important. This is a way that you can use to find out if the inertia or desperation are taking effects on you. Anyway we are not perfect, so you may also mark the piece of copied code (as you like) in order that you remember to check it when something happens.
Desperate debugging errors
Everyone has desperate, at least, once in his life. If you ever have programmed something it should also include debugging as well. There are some activities that are performed during debugging, one of those (discouraged) activities is changing the variable values in order to find out any reaction. This is usually done in environments without many debugging tools. In those cases that is pretty useful. However, if you act desperately you are more likely to keep it like that, making the program to throw errors every time. For example let us say you want to debug something like this:
//var thing = getValueFromSomewhere(); //this line was commented while debugging
var thing = true;
//BEGIN CODE BLOCK
//let us say you are debugging this piece of code and that was the reason
//you had to change the variable value above, but if you keep it as is,
//while being desperate, you are more likely to have problems later
if(thing){
//code
}else{
//code
}
//END CODE BLOCK
As you may notice, if you do not turn back the variable as it was once you have figured out what was happening while being desperate. You are very likely to lose a really valuable time in that simple thing. there are more things like this that people usually do in order to debug programs (in that kind of situations). Such as overriding the security of the system or replacing the files, but remember that all these things along with desperation may always generate problems.
Solution
Debugging something fast is something really hard to do because if you ever desperate you will produce errors instead of fixing them (my personal opinion). As you may notice, I have been saying the word “desperation” for sometime. So the first thing to do is staying calmed. Additionally to that, you can add some comments to the lines that you are debugging so you may notice if there are still lines to be changed, it would be something like this;
/*var thing = getValueFromSomewhere(); //DEBUG*/ var thing = true;
if(thing){
//code
}else{
//code
}
As I said, people usually start doing discouraged activities when desperate such as changing files. overriding the security measures, and more, especially in case that you do not have a real debugger. With this you can know at once if you are debugging something manually. The most important thing is being aware of the things you are doing and using the proper tools. Always remember to revert these changes, and never perform this kind of changes in a production environment.
Platform specific errors
Although most of these mistakes can be committed on any platform. There are some simple mistakes that can be committed on specific platforms/systems/programs/tools. In most of cases there is no way to debug them fast (at least on your own) and there is no way that you can avoid this simply because you do not know what you do not know. That is why some project require some experience in order to meet deadlines at time. Interestingly this happens even if the errors are really simple.
“UTF8 BOM” codification (PHP error)
As you may know, PHP is an interpreted programming languages optimized for the web. It usually works with a web server, PHP usually execute the code of every text file indicated by the server (usually *.php
files) that is enclosed by these tags; <?php
& ?>
and the rest of the text is sent to the server (usually in HTML format, it depends on the server). the PHP capabilities also include managing the HTTP headers sent to the client (actually, this is how we set the format of the rest of the text). These headers are pieces of data representing information about the request sent and they must be sent before the content of the request, if not it would throw an error. Well, the “UTF8 BOM” text format usually writes an invisible character at the beginning of the file. If you use this format in one of your PHP files this invisible character will be interpret as HTML (or whatever mimetype you are using) and is very likely to be sent before the HTTP headers throwing an error.
//if you are using the "UTF8 BOM" codification although this code is OK,
//it won't work until you change it in the text editor.
//there is supposed to be an invisible character here
//which is being shown before the session start (thanks to "UTF8 BOM"),
//the sessions start will require to access the header but it will fail
//because of that invisible character.
<?php
session_start();
...
?>
This is a really simple error that may take you hours if you are not warned of this before (I hope you know it now). The way to solve this simple problem is to change the text format to another format in the text editor (or IDE, I do not know what you are using).
Server time configuration
I said that some platforms may have specific ways to work. Well, in some cases some platforms may check the hours, dates, timestamps and more. In an Amazon WebService (SOAP) api there is a limitation with the time. It must be perfectly configured in the server because if not the connection will not be established. It seems pretty simple to know, however, while working with a client I had to perform some little tasks including one related with Amazon SOAP api. The official time of Venezuela was changed from -4:00 UTC to -4:30 UTC, but some servers (who sync the time zones automatically) still have “-4:00 UTC” as Venezuela’s time so everything what I did was leading to an error anyway. If you are not aware of these kind of things you may spend hours (as me) figuring out why is not your code working properly.
Solution
As I said, these errors can not be solved easily on your own, but you might get a great help if you participate on forums (e.g.: stackoverflow.com [my favorite]). You may also want to read blog entries related to the problem that you have, If you can find someone who can help you out with the problem that may also help, but if you really want to expedite the debugging process, it is highly encouraged that you contact someone else in order to get the help that you need (because in those cases you need help). I really encourage using forums. I think is the best option available.
Version errors
Sometimes it is not your fault entirely. Sometimes it may depend on something else such as the version of the program/system/platform/tool you are using. This is not a platform specific error is just the version you are using. This means that you will not be able to fix the error until you notice that the version of the program/system/platform/tool you are using has some problems. Because you are very likely to waste time on such a simple error (if you have no previous experience, of course). This might be considered as a mistake. For example, let us review this situations (based on a real experience); you want to consume a SOAP Web service with PHP, let us say you are going to use NuSOAP because it is pretty simple to use, but when trying to consume the service you suddenly get an error. Then you debug the code for hours trying to figure out what is wrong with it without success until you notice you were using PHP 5.2.8. In those cases it is encouraged that you check the version of the programs because some of them may be making the code fail.
Solution
As I said, there is no apparent solution. In those cases when you feel you are not capable to handle the situations. Think about these kind of things, try changing the programs if possible, for example try using the SOAP library of PHP instead of NuSOAP, try using NGINX or LIGHTTPD instead of APACHE, try using another OS. If it works you know very well that the error is in the program you are using.
Remember that…
Debugging is not easy:
Remember that debugging is one of the hardest activities in computer science (my personal opinion). If you can do it fast then good for you, but if you can not try not to feel bad about it. Try to ask for help if possible, also remember that we are not perfect and we always make mistakes.
Being careful with desperation and inertia:
As you may notice, I have mentioned several times the words “inertia” and “desperation”. These are the based of many problems that you may have. If you do not calm yourself and take back the control. You are more likely to make even more mistakes.
Being careful with the tools you use:
This is really important, while working try to use tools that really help you (not just teach you). Let me give you an example, A long time before, I used to use tool without many automation features such as the MySQL client instead of phpmyadmin, notepad++ and GCC instead of any IDE. This can be more didactic but not useful and it may take you more time than the time you may take using a really useful tool.
Practice and experience is the only warranty:
There is no warranty for short debugging times or fixes for the mistakes you may commit but the experience itself. That is the only way (please do not be scared about it), so calm down and try to fix it by yourself (if it is possible). Remember the next time it happens you will be prepared to handle it, so try to fix as many errors/mistakes as you can, by time you will become more skilled.